One common problem in Revit models is to prioritize the resolution of the warnings are shown under Manage > Inquiry > Review Warnings. These error messages are generated, with or without knowledge from the users, when working in the project and mostly ignored until they are a big problem that affects the performance and integrity of the Revit model.
Some of these warnings are not critical errors like Highlighted elements are joined but do not intersect, but others like Highlighted walls overlap can decrease the model’s performance and induce wrong schedules and material takeoffs. To prioritize the time spent in fixing these warnings, a system of warning levels has to be in place. That is what we are going to address in this post and the next one.
Extract all warnings from a Revit project
The first step to get a warning classification system is to extract all possible warnings in Revit and prioritize them in a master file. We will use this master file to check against warning matches in our project models. For this purpose, we are going to use this Revit macro:
/* Import
using System.IO;
using System.Text;
*/
public void RetrieveAllWarnings()
{
// Store warnings
var warningsText = new StringBuilder();
// Retrieve all failures
FailureDefinitionRegistry failDefRegistry = Autodesk.Revit.ApplicationServices.Application.GetFailureDefinitionRegistry();
List<FailureDefinitionAccessor> allFailures = failDefRegistry.ListAllFailureDefinitions() as List<FailureDefinitionAccessor>;
foreach (var failure in allFailures)
{
// Filter all warnings from document
if (failure.GetSeverity() == FailureSeverity.Warning)
{
warningsText.AppendLine(failure.GetDescriptionText()).AppendLine();
}
}
// Path to file to store all warnings
string warningsFile = Environment.GetFolderPath(Environment.SpecialFolder.Desktop) + @"\All Warnings.txt";
// Check if file exists and create new file to store warnings
if (!File.Exists(warningsFile))
{
using (StreamWriter sw = new StreamWriter(warningsFile))
{
sw.Write(warningsText.ToString());
}
}
else
{
TaskDialog.Show("Error", "File already exist");
}
}
It will export all the possible warnings Revit can yield into a txt file located in your Desktop.
Classify all possible Revit warnings
Now it comes the hard part, how do we sort the warnings into a prioritized list? Well, the bad news is that we have to do it manually. And the good news is that I have done it for you in this file:
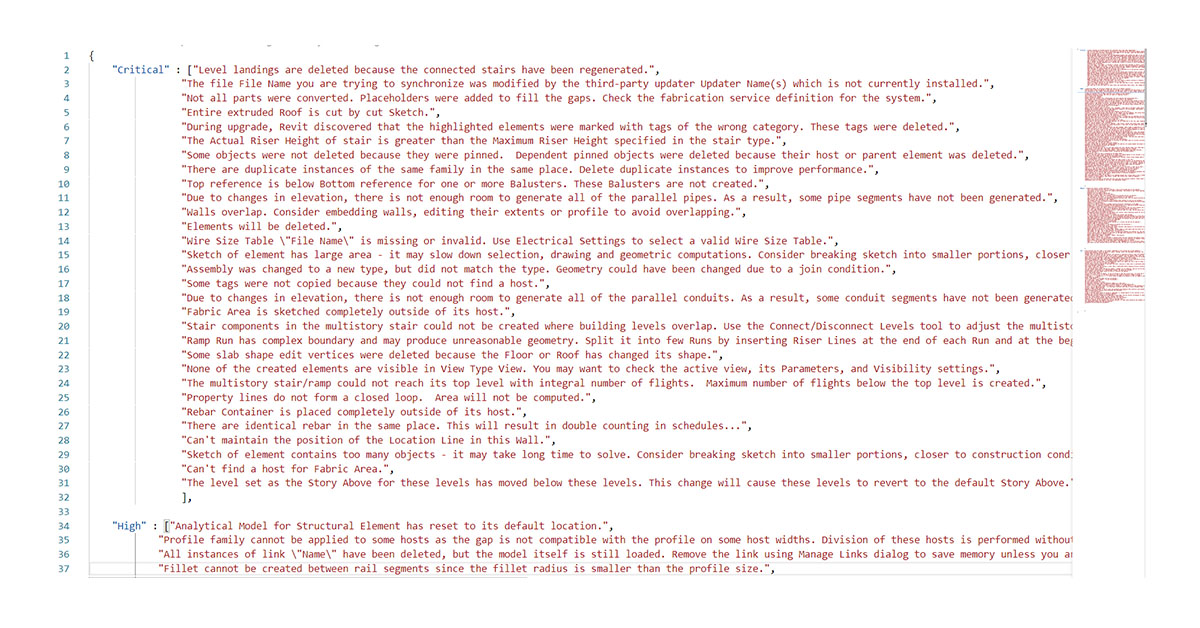
It may not have all your desired classification and levels, but with some small changes, you will find it tailored to your needs rather than classifying all the warnings from scratch. So, if you make some nice improvements, don’t forget to place a pull request!
Now, let’s go for the next post that shows how it is used.
Leave a Reply